ダイアログ表示を行うビューモデル(その3)の続きです。
前回まででライブラリが完成したので、今回はライブラリを使ったアプリケーション例です。今回は「プロジェクトの作成」「プロジェクトへの設定」「ビューモデル」を書きます。ビュー関係は次回書く予定です。
最初に画面の説明から。
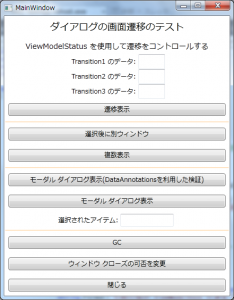
「遷移表示」ボタンまでが一区切りで、MainWindow -> TransitionWindow1 -> TransitionWindow2 -> TransitionWindow3 でデータ入力が完了して MainWindow に戻って、それぞれの画面で入力れた値が表示されるという画面遷移になっています。
「選択後に別ウィンドウ」ボタンは、SelectWindow に遷移して ComboBox の選択を行い「OK」ボタンをクリックすると遷移が完了し、その後新たな画面遷移で MainWindow から AfterSelectWindow に遷移して選択された値を表示するという画面遷移になっています。 AfterSelectWindow を閉じると MainWindow に戻ります。
「複数表示」ボタンは、画面遷移は行わずにモードレス ウィンドウを追加表示します。追加表示するウィンドウは、最初クローズ禁止状態になっていて、当該ウィンドウの「閉じる」ボタンは無効になっています。この状態で MainWindow の「閉じる」ボタンをクリックすると「閉じることのできないウィドウがあります。」というメッセージが表示されて、MainWindow のクローズは行われません。追加表示されたウィンドウの「ウィンドウ クローズの可否を変更」ボタンをクリックすると、クローズ禁止状態の On/Off が行えます。
「モーダル ダイアログ表示(DataAnnotationsを利用した検証)」と「モーダル ダイアログ表示」ボタンは、モーダル ダイアログを表示します。前者は DataAnnotationsを利用した検証を行なって検証エラーの有無で「OK」ボタンの有効/無効を制御する例(ライブラリのデフォルト機能)で、後者はビューモデル側で有効/無効を返すメソッドをオーバーライドする例になっています。
「GC」ボタンはガベージコレクションを強制的に行います。ビューモデルで Dispose メソッドをオーバーライドしてデバッグ出力にメッセージを表示するようにしているので、ビューモデルの破棄を確認できます。
「ウィンドウ クローズの可否を変更」ボタンは、MainWindow のクローズ禁止状態の On/Off を行います。クローズ禁止状態になると MainWindow の「閉じる」ボタンが無効になるだけですが、この状態で OS のログオフを行うと、OS からのアプリケーション シャットダウン時に当該アプリケーション側で「閉じることのできないウィンドウがあります。強制的に閉じてもよろしいですか(保存していないデータなどは破棄されます)?”」というメッセージが出ることを確認できます(このロジックは App.Xaml(Application の SessionEnding プロパティの指定) と App.Xaml.cs(イベント ハンドラ のメソッド) に記述しています)。
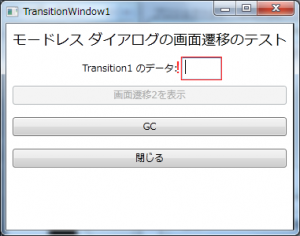
画面遷移の1番目の画面です。
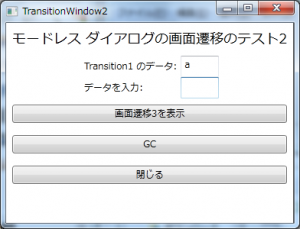
画面遷移の2番目の画面です。
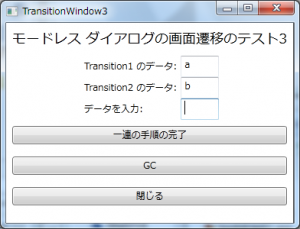
画面遷移の3番目の画面です。
「一連の手続きの完了」ボタンで MainWindow に戻って入力された値を表示します。
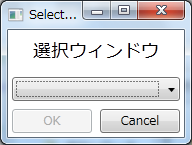
「選択後に別ウィンドウ」の選択画面です。
「OK」ボタンクリックで選択が完了します。
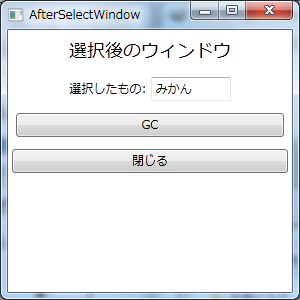
「選択後に別ウィンドウ」の選択後に表示する画面です。
「閉じる」ボタンクリックで MainWindow に戻ります。
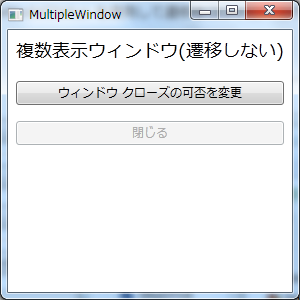
「複数表示」で表示する画面です。
「ウィンドウ クローズの可否を変更」ボタンクリックで当該ウィンドウのクローズ禁止状態の On/Off が行えます。
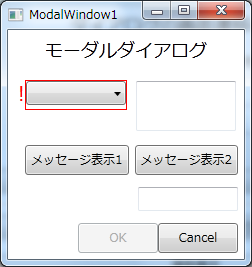
「モーダル ダイアログ表示(DataAnnotationsを利用した検証)」で表示する画面です。
「メッセージ表示1」「メッセージ表示2」のボタンは、2つのボタンを持つ MessageBox を表示し、クリックされたボタンに応じて TextBox にメッセージを表示します(MessageBox の表示とユーザー応答に対応する処理の例になっています)。
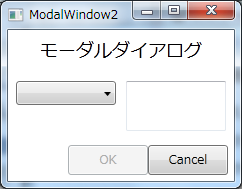
「モーダル ダイアログ表示」で表示する画面です。
次にプログラムです。
WPF のプロジェクトを作成します(プロジェクト名は「TestApp」にしています)。
対象のフレームワークは、.NET Framework 4.5 にしてください。
まずは必要な DLL ファイルへの参照を追加します。
- System.ComponentModel.DataAnnotations
- TransitionViewModelBase
- System.Windows.Interactivity (BlendSDK)
- Microsoft.Expression.Interactions (BlendSDK)
次にビューモデル関連です。
プロジェクトに「ViewModels」フォルダを作成し、ViewModels フォルダ下に「Container」フォルダを作成します。
Container フォルダに「SelectContainer」クラスを作成します。
using MakCraft.ViewModels;
namespace TestApp.ViewModels.Container
{
public class SelectContainer : TransitionContainerBase
{
public SelectContainer(string key, TransitionViewModelBase viewModel) : base(key, viewModel)
{
}
public string ItemName { get; set; }
}
}
続けて「TransitionContainer」クラスを作成します。
using MakCraft.ViewModels;
namespace TestApp.ViewModels.Container
{
public class TransitionContainer : TransitionContainerBase
{
public TransitionContainer(string key, TransitionViewModelBase viewModel) : base(key, viewModel)
{
}
public string Transition1Text { get; set; }
public string Transition2Text { get; set; }
public string Transition3Text { get; set; }
}
}
次に ViewModels フォルダに「AfterSelectWindowViewModel」クラスを作成します。
using System;
using System.Linq;
using System.Windows.Input;
using MakCraft.ViewModels;
using TestApp.ViewModels.Container;
namespace TestApp.ViewModels
{
class AfterSelectWindowViewModel : TransitionViewModelBase
{
public AfterSelectWindowViewModel()
{
}
private string _selectedItem;
public string SelectedItem
{
get { return _selectedItem; }
set
{
_selectedItem = value;
base.RaisePropertyChanged(() => SelectedItem);
}
}
private void completeExecute()
{
base.TransitionComplete();
}
private ICommand _completeCommand;
public ICommand CompleteCommand
{
get
{
if (_completeCommand == null)
_completeCommand = new RelayCommand(completeExecute);
return _completeCommand;
}
}
private void gcExecute()
{
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
}
private ICommand _gcCommand;
public ICommand GcCoomand
{
get
{
if (_gcCommand == null)
_gcCommand = new RelayCommand(gcExecute);
return _gcCommand;
}
}
// MainWindowViewModel から渡されてきたデータをセット
protected override void OnContainerReceived(object container)
{
SelectedItem = ((SelectContainer)container).ItemName;
base.OnContainerReceived(container);
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** AfterSelectWindowViewModel disposed.");
base.Dispose(disposing);
}
}
}
続けて「MainWindowViewModel」クラスを作成します。
using System;
using System.Windows.Input;
using MakCraft.ViewModels;
using MakCraft.Behaviors.Interfaces;
using TestApp.ViewModels.Container;
namespace TestApp.ViewModels
{
class MainWindowViewModel : TransitionViewModelBase
{
public MainWindowViewModel() { }
private string _trans1Text;
public string Trans1Text
{
get { return _trans1Text; }
set
{
_trans1Text = value;
base.RaisePropertyChanged(() => Trans1Text);
}
}
private string _trans2Text;
public string Trans2Text
{
get { return _trans2Text; }
set
{
_trans2Text = value;
base.RaisePropertyChanged(() => Trans2Text);
}
}
private string _trans3Text;
public string Trans3Text
{
get { return _trans3Text; }
set
{
_trans3Text = value;
base.RaisePropertyChanged(() => Trans3Text);
}
}
private string _selectedBook;
public string SelectedBook
{
get { return _selectedBook; }
set
{
_selectedBook = value;
base.RaisePropertyChanged(() => SelectedBook);
}
}
private object _modelessKick;
/// <summary>
/// モードレスダイアログの表示のキック用
/// </summary>
public object ModelessKick
{
get { return _modelessKick; }
set
{
_modelessKick = value;
base.RaisePropertyChanged(() => ModelessKick);
}
}
private object _modalKick;
/// <summary>
/// モーダルダイアログの表示のキック用
/// </summary>
public object ModalKick
{
get { return _modalKick; }
set
{
_modalKick = value;
base.RaisePropertyChanged(() => ModalKick);
}
}
private void gcExecute()
{
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
}
private ICommand _gcCommand;
public ICommand GcCoomand
{
get
{
if (_gcCommand == null)
_gcCommand = new RelayCommand(gcExecute);
return _gcCommand;
}
}
private void showTransitionWindowExecute()
{
Trans1Text = string.Empty;
Trans2Text = string.Empty;
Trans3Text = string.Empty;
// 遷移を行う画面を表示
base.DialogType = typeof(TransitionWindow1);
var container = new TransitionContainer(transitionName.trans.ToString(), this);
base.CommunicationDialog = container;
ModelessKick = new object();
base.DisplayMode = WindowAction.Hide;
}
private ICommand _showTransitionWindowCommand;
public ICommand ShowTransitionWindowCommand
{
get
{
if (_showTransitionWindowCommand == null)
_showTransitionWindowCommand = new RelayCommand(showTransitionWindowExecute);
return _showTransitionWindowCommand;
}
}
private void showTransAfterSelectedExecute()
{
// 遷移を行う画面を表示
// A -> B 完了 -> A を経由して C 表示のパターン
// C の表示は OnFinished メソッド側で行っている
base.DialogType = typeof(SelectWindow);
var container = new SelectContainer(transitionName.select.ToString(), this);
base.CommunicationDialog = container;
ModelessKick = new object();
base.DisplayMode = WindowAction.Hide;
}
private ICommand _showTransAfterSelectedCommand;
public ICommand ShowTransAfterSelectedCommand
{
get
{
if (_showTransAfterSelectedCommand == null)
_showTransAfterSelectedCommand = new RelayCommand(showTransAfterSelectedExecute);
return _showTransAfterSelectedCommand;
}
}
private void showMultipleWindowExecute()
{
base.DialogType = typeof(MultipleWindow);
base.CommunicationDialog = null;
ModelessKick = new object();
}
private ICommand _showMultipleWindowCommand;
public ICommand ShowMultipleWindowCommand
{
get
{
if (_showMultipleWindowCommand == null)
_showMultipleWindowCommand = new RelayCommand(showMultipleWindowExecute);
return _showMultipleWindowCommand;
}
}
private void showModalWindowExecute()
{
SelectedBook = string.Empty;
base.DialogType = typeof(ModalWindow1);
base.CommunicationDialog = null;
base.DialogActionCallback = dialogResult =>
{
if (dialogResult.HasValue && dialogResult.Value)
{
var viewModel = base.ResultViewModel as ModalWindow1ViewModel;
SelectedBook = viewModel.SelectedBook.Title;
}
};
ModalKick = new object();
}
private ICommand _showModalWindowCommand;
public ICommand ShowModalWindowCommand
{
get
{
if (_showModalWindowCommand == null)
_showModalWindowCommand = new RelayCommand(showModalWindowExecute);
return _showModalWindowCommand;
}
}
private void showModalWindow2Execute()
{
SelectedBook = string.Empty;
base.DialogType = typeof(ModalWindow2);
base.CommunicationDialog = null;
base.DialogActionCallback = dialogResult =>
{
if (dialogResult.HasValue && dialogResult.Value)
{
var viewModel = base.ResultViewModel as ModalWindow2ViewModel;
SelectedBook = viewModel.SelectedBook.Title;
}
};
ModalKick = new object();
}
private ICommand _showModalWindow2Command;
public ICommand ShowModalWindow2Command
{
get
{
if (_showModalWindow2Command == null)
_showModalWindow2Command = new RelayCommand(showModalWindow2Execute);
return _showModalWindow2Command;
}
}
private void changeClosableExecute()
{
_isClosable = !_isClosable;
}
private ICommand _changeClosableCommand;
public ICommand ChangeClosableCommand
{
get
{
if (_changeClosableCommand == null)
_changeClosableCommand = new RelayCommand(changeClosableExecute);
return _changeClosableCommand;
}
}
// 画面遷移の一連の手順の完了時に行う処理
public override void OnFinished(ITransContainer container)
{
switch ((transitionName)Enum.Parse(typeof(transitionName), container.Key))
{
case transitionName.trans:
var trans = container as TransitionContainer;
Trans1Text = trans.Transition1Text;
Trans2Text = trans.Transition2Text;
Trans3Text = trans.Transition3Text;
break;
case transitionName.select:
// 遷移を行う画面を表示
base.DialogType = typeof(AfterSelectWindow);
var afterContainer = new SelectContainer(transitionName.after.ToString(), this);
afterContainer.ItemName = ((SelectContainer)container).ItemName;
base.CommunicationDialog = afterContainer;
ModelessKick = new object();
base.DisplayMode = WindowAction.Hide;
break;
case transitionName.after:
break;
}
base.OnFinished(container);
}
private bool _isClosable = true;
protected override bool WindowCloseCanExecute(object param)
{
return _isClosable;
}
public override void WindowClose()
{
try
{
ViewModelUtility.CloseViewModels(typeof(MultipleWindowViewModel));
}
catch (WindowPendingProcessException)
{
// MessageDialogAction ビヘイビアを使用してメッセージボックスを出す
base.MessageDialogActionParam = new MessageDialogActionParameter("閉じることのできないウィンドウがあります。",
"クローズできません");
base.RaisePropertyChanged(() => MessageDialogActionParam);
return;
}
base.WindowClose();
}
private enum transitionName
{
trans,
select,
after,
}
}
}
続けて「ModalWindow1ViewModel」クラスを作成します。
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Windows;
using System.Windows.Input;
using MakCraft.ViewModels;
namespace TestApp.ViewModels
{
class ModalWindow1ViewModel : ModalViewModelBase
{
public ModalWindow1ViewModel()
{
setup();
}
private List<Book> _books;
public List<Book> Books
{
get { return _books; }
set
{
_books = value;
base.RaisePropertyChanged(() => Books);
}
}
private Book _selectedBook;
[Required(ErrorMessage = "選択してください。")]
public Book SelectedBook
{
get { return _selectedBook; }
set
{
_selectedBook = value;
base.RaisePropertyChanged(() => SelectedBook);
}
}
private string _messageText;
public string MessageText
{
get { return _messageText; }
set
{
_messageText = value;
base.RaisePropertyChanged(() => MessageText);
}
}
private void messageExecute()
{
MessageText = string.Empty;
// コールバックの処理を設定
base.MessageDialogActionCallback = result =>
{
switch (result)
{
case MessageBoxResult.Yes:
MessageText = "Yes が選択されました。";
break;
case MessageBoxResult.No:
MessageText = "No が選択されました。";
break;
}
};
// MessageDialogAction ビヘイビアを使用してメッセージボックスを出す
base.MessageDialogActionParam = new MessageDialogActionParameter("クリックされました。",
"メッセージ", MessageBoxButton.YesNo, true);
base.RaisePropertyChanged(() => MessageDialogActionParam);
}
private ICommand _messageCommand;
public ICommand MessageCommand
{
get
{
if (_messageCommand == null)
_messageCommand = new RelayCommand(messageExecute);
return _messageCommand;
}
}
private void message2Execute()
{
MessageText = string.Empty;
// コールバックの処理を設定
base.MessageDialogActionCallback = result =>
{
switch (result)
{
case MessageBoxResult.OK:
MessageText = "OK が選択されました。";
break;
case MessageBoxResult.Cancel:
MessageText = "Cancel が選択されました。";
break;
}
};
// MessageDialogAction ビヘイビアを使用してメッセージボックスを出す
base.MessageDialogActionParam = new MessageDialogActionParameter("クリックされました。",
"メッセージ", MessageBoxButton.OKCancel, true);
base.RaisePropertyChanged(() => MessageDialogActionParam);
}
private ICommand _message2Command;
public ICommand Message2Command
{
get
{
if (_message2Command == null)
_message2Command = new RelayCommand(message2Execute);
return _message2Command;
}
}
private void setup()
{
Books = new List<Book> {
new Book { Title = "猫の本", Note = "著者: みけ\r\n出版社: 猫の出版社\r\n概要: 猫ねこネコ" },
new Book { Title = "犬の本", Note = "著者: ぽち\r\n出版社: 犬の出版社\r\n概要: 犬いぬイヌ" },
new Book { Title = "金魚の本", Note = "著者: きん\r\n出版社: 金魚の出版社\r\n概要: 金魚きんぎょキンギョ" },
new Book { Title = "鳥の本", Note = "著者: ぴよ\r\n出版社: 鳥の出版社\r\n概要: 鳥とりトリ" },
};
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** ModalWindow1ViewModel disposed.");
base.Dispose(disposing);
}
}
public class Book
{
public string Title { get; set; }
public string Note { get; set; }
}
}
続けて「ModalWindow2ViewModel」クラスを作成します。
using System.Collections.Generic;
using MakCraft.ViewModels;
namespace TestApp.ViewModels
{
class ModalWindow2ViewModel : ModalViewModelBase
{
public ModalWindow2ViewModel()
{
setup();
}
private List<Book> _books;
public List<Book> Books
{
get { return _books; }
set
{
_books = value;
base.RaisePropertyChanged(() => Books);
}
}
private Book _selectedBook;
public Book SelectedBook
{
get { return _selectedBook; }
set
{
_selectedBook = value;
base.RaisePropertyChanged(() => SelectedBook);
}
}
private void setup()
{
Books = new List<Book> {
new Book { Title = "猫の本", Note = "著者: みけ\r\n出版社: 猫の出版社\r\n概要: 猫ねこネコ" },
new Book { Title = "犬の本", Note = "著者: ぽち\r\n出版社: 犬の出版社\r\n概要: 犬いぬイヌ" },
new Book { Title = "金魚の本", Note = "著者: きん\r\n出版社: 金魚の出版社\r\n概要: 金魚きんぎょキンギョ" },
new Book { Title = "鳥の本", Note = "著者: ぴよ\r\n出版社: 鳥の出版社\r\n概要: 鳥とりトリ" },
};
}
protected override bool OkCanExecute(object param)
{
return (SelectedBook != null);
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** ModalWindow2ViewModel disposed.");
base.Dispose(disposing);
}
}
}
続けて「MultipleWindowViewModel」クラスを作成します。
using System;
using System.Windows.Input;
using MakCraft.ViewModels;
namespace TestApp.ViewModels
{
class MultipleWindowViewModel : TransitionViewModelBase
{
public MultipleWindowViewModel() { }
private void changeClosableExecute()
{
_isClosable = !_isClosable;
}
private ICommand _changeClosableCommand;
public ICommand ChangeClosableCommand
{
get
{
if (_changeClosableCommand == null)
_changeClosableCommand = new RelayCommand(changeClosableExecute);
return _changeClosableCommand;
}
}
protected override void Dispose(bool disposing)
{
Console.WriteLine("** MultipleWindowViewModel disposed.");
base.Dispose(disposing);
}
private bool _isClosable = false;
protected override bool WindowCloseCanExecute(object param)
{
return _isClosable;
}
}
}
続けて「SelectWindowViewModel」クラスを作成します。
using System.Collections.Generic;
using System.Windows.Input;
using MakCraft.ViewModels;
using TestApp.ViewModels.Container;
namespace TestApp.ViewModels
{
class SelectWindowViewModel : TransitionViewModelBase
{
public SelectWindowViewModel()
{
Items = initList();
}
private List<string> _items;
public List<string> Items
{
get { return _items; }
set
{
_items = value;
base.RaisePropertyChanged(() => Items);
}
}
private string _selectedItem;
public string SelectedItem
{
get { return _selectedItem; }
set
{
_selectedItem = value;
base.RaisePropertyChanged(() => SelectedItem);
}
}
protected override void TransitionComplete()
{
((SelectContainer)base.Container).ItemName = SelectedItem;
base.TransitionComplete();
}
private void completeExecute()
{
TransitionComplete();
}
private bool completeCanExecute(object param)
{
return !string.IsNullOrEmpty(_selectedItem);
}
private ICommand _completeCommand;
public ICommand CompleteCommand
{
get
{
if (_completeCommand == null)
_completeCommand = new RelayCommand(completeExecute, completeCanExecute);
return _completeCommand;
}
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** SelectWindowViewModel disposed.");
base.Dispose(disposing);
}
private List<string> initList()
{
return new List<string> {
"りんご", "柿", "ぶどう", "みかん"
};
}
}
}
続けて「TransitionWindow1ViewModel」クラスを作成します。
using System;
using System.ComponentModel.DataAnnotations;
using System.Windows.Input;
using MakCraft.Behaviors.Interfaces;
using MakCraft.ViewModels;
using TestApp.ViewModels.Container;
namespace TestApp.ViewModels
{
class TransitionWindow1ViewModel : TransitionViewModelBase
{
public TransitionWindow1ViewModel()
{
}
private string _trans1Text;
[Required(ErrorMessage = "この項目は必須項目です。")]
public string Trans1Text
{
get { return _trans1Text; }
set
{
_trans1Text = value;
base.RaisePropertyChanged(() => Trans1Text);
}
}
private object _modelessKick;
/// <summary>
/// モードレスダイアログの表示のキック用
/// </summary>
public object ModelessKick
{
get { return _modelessKick; }
set
{
_modelessKick = value;
base.RaisePropertyChanged(() => ModelessKick);
}
}
private void showTransitionWindow2Execute()
{
base.DialogType = typeof(TransitionWindow2);
// コンテナに前画面のビューモデルを記録
((TransitionContainer)base.Container).PreviousViewModel = this;
((TransitionContainer)base.Container).Transition1Text = _trans1Text;
base.CommunicationDialog = base.Container;
ModelessKick = new object();
base.DisplayMode = WindowAction.Hide;
}
private bool showTransitionWindow2CanExecute(object param)
{
return base.IsValid;
}
private ICommand _showTransitionWindow2Command;
public ICommand ShowTransitionWindow2Command
{
get
{
if (_showTransitionWindow2Command == null)
_showTransitionWindow2Command = new RelayCommand(showTransitionWindow2Execute, showTransitionWindow2CanExecute);
return _showTransitionWindow2Command;
}
}
private void gcExecute()
{
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
}
private ICommand _gcCommand;
public ICommand GcCoomand
{
get
{
if (_gcCommand == null)
_gcCommand = new RelayCommand(gcExecute);
return _gcCommand;
}
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** TransitionWindowViewModel1 disposed.");
base.Dispose(disposing);
}
}
}
続けて「TransitionWindow2ViewModel」クラスを作成します。
using System;
using System.Windows.Input;
using MakCraft.Behaviors.Interfaces;
using MakCraft.ViewModels;
using TestApp.ViewModels.Container;
namespace TestApp.ViewModels
{
class TransitionWindow2ViewModel : TransitionViewModelBase
{
public TransitionWindow2ViewModel()
{
}
private string _trans1Text;
public string Trans1Text
{
get { return _trans1Text; }
set
{
_trans1Text = value;
base.RaisePropertyChanged(() => Trans1Text);
}
}
private string _trans2Text;
public string Trans2Text
{
get { return _trans2Text; }
set
{
_trans2Text = value;
base.RaisePropertyChanged(() => Trans2Text);
}
}
private object _modelessKick;
/// <summary>
/// モードレスダイアログの表示のキック用
/// </summary>
public object ModelessKick
{
get { return _modelessKick; }
set
{
_modelessKick = value;
base.RaisePropertyChanged(() => ModelessKick);
}
}
private void showTransitionWindow3Execute()
{
base.DialogType = typeof(TransitionWindow3);
// コンテナに前画面のビューモデルを記録
((TransitionContainer)base.Container).PreviousViewModel = this;
((TransitionContainer)base.Container).Transition2Text = _trans2Text;
base.CommunicationDialog = base.Container;
ModelessKick = new object();
base.DisplayMode = WindowAction.Hide;
}
private bool showTransitionWindow3CanExecute(object param)
{
return !string.IsNullOrEmpty(Trans2Text);
}
private ICommand _showTransitionWindow3Command;
public ICommand ShowTransitionWindow3Command
{
get
{
if (_showTransitionWindow3Command == null)
_showTransitionWindow3Command = new RelayCommand(
showTransitionWindow3Execute,
showTransitionWindow3CanExecute);
return _showTransitionWindow3Command;
}
}
private void gcExecute()
{
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
}
private ICommand _gcCommand;
public ICommand GcCoomand
{
get
{
if (_gcCommand == null)
_gcCommand = new RelayCommand(gcExecute);
return _gcCommand;
}
}
// Transition1ViewModelBase から渡されてきたデータをセット
protected override void OnContainerReceived(object container)
{
Trans1Text = ((TransitionContainer)container).Transition1Text;
base.OnContainerReceived(container);
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** TransitionWindowViewModel2 disposed.");
base.Dispose(disposing);
}
}
}
最後に「TransitionWindow3ViewModel」クラスを作成します。
using System;
using System.Windows.Input;
using MakCraft.ViewModels;
using TestApp.ViewModels.Container;
namespace TestApp.ViewModels
{
class TransitionWindow3ViewModel : TransitionViewModelBase
{
public TransitionWindow3ViewModel()
{
}
private string _trans1Text;
public string Trans1Text
{
get { return _trans1Text; }
set
{
_trans1Text = value;
base.RaisePropertyChanged(() => Trans1Text);
}
}
private string _trans2Text;
public string Trans2Text
{
get { return _trans2Text; }
set
{
_trans2Text = value;
base.RaisePropertyChanged(() => Trans2Text);
}
}
private string _trans3Text;
public string Trans3Text
{
get { return _trans3Text; }
set
{
_trans3Text = value;
base.RaisePropertyChanged(() => Trans3Text);
}
}
// 完了時の処理
protected override void TransitionComplete()
{
((TransitionContainer)base.Container).Transition3Text = _trans3Text;
base.TransitionComplete();
}
// 完了コマンド
private void completeExecute()
{
TransitionComplete();
}
private bool completeCanExecute(object param)
{
return !string.IsNullOrEmpty(Trans3Text);
}
private ICommand _completeCommand;
public ICommand CompleteCommand
{
get
{
if (_completeCommand == null)
_completeCommand = new RelayCommand(completeExecute, completeCanExecute);
return _completeCommand;
}
}
private void gcExecute()
{
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
}
private ICommand _gcCommand;
public ICommand GcCoomand
{
get
{
if (_gcCommand == null)
_gcCommand = new RelayCommand(gcExecute);
return _gcCommand;
}
}
// Transition1ViewModelBase から渡されてきたデータをセット
protected override void OnContainerReceived(object container)
{
var tContainer = container as TransitionContainer;
Trans1Text = tContainer.Transition1Text;
Trans2Text = tContainer.Transition2Text;
base.OnContainerReceived(container);
}
protected override void Dispose(bool disposing)
{
System.Diagnostics.Debug.WriteLine("** TransitionWindowViewModel3 disposed.");
base.Dispose(disposing);
}
}
}
以上でビューモデルは完成です。
ビュー関係は、次回に書く予定です。
「ダイアログ表示を行うビューモデル(その4)」への2件のフィードバック